When working with programming languages such as PHP, developers occasionally encounter perplexing errors. One such error is “error call to a member function getCollectionParentId() on null”, which can stall your progress if not addressed properly. This article explores the underlying causes, solutions, and preventive measures for this error in an approachable and organized way.
What Does This Error Mean?
The “error call to a member function getCollectionParentId() on null” signifies that the code is attempting to invoke the getCollectionParentId()
function on an object that doesn’t exist (null). In simple terms, the program expects an object but finds nothing. As a result, it cannot perform the desired operation.
This issue is common in object-oriented programming (OOP), where functions (methods) operate on objects. If the object is null, the program cannot execute the intended function.
Common Causes of the Error
Understanding why this error arises is crucial to effectively resolving it. Here are the most frequent reasons:
1. Null Object Reference
One of the primary culprits is trying to use a method on a null object. For instance, if the code expects an object but receives a null value instead, the error occurs.
- Example in PHP:phpCopy code
$object = null; $parentId = $object->getCollectionParentId(); // This will trigger the error
2. Incorrect Object Initialization
An object might not be properly instantiated or its dependencies might not be set up correctly.
- Example: If a function responsible for creating an object returns null due to a misconfiguration, calling any method on the object will throw this error.
3. Early Returns or Uncaught Exceptions
Errors during execution might prevent an object from being fully initialized. If the program logic leads to an early return or uncaught exception, subsequent attempts to call methods on the object will fail.
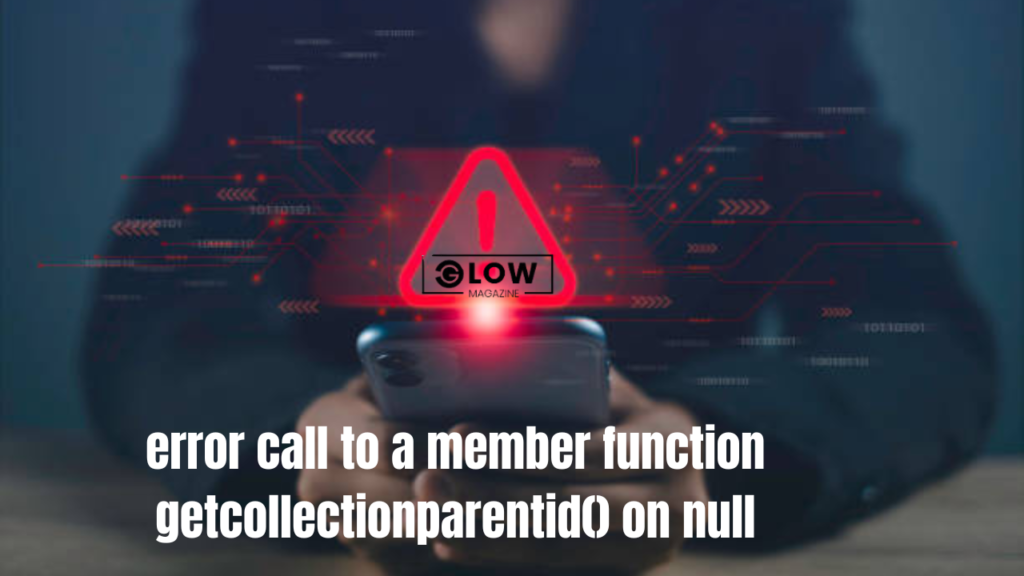
4. Timing Issues in Asynchronous Operations
When dealing with asynchronous tasks, such as fetching data from a database or API, the object may not be fully ready when accessed.
- Example: If a promise or callback hasn’t resolved when the method is invoked, the object will still be null.
How to Resolve the Error
1. Perform Null Checks
Before calling the method, ensure the object is not null. This approach minimizes unexpected crashes.
- Solution in PHP:phpCopy code
if ($object !== null) { $parentId = $object->getCollectionParentId(); } else { echo "The object is null."; }
2. Verify Object Initialization
Ensure the object is correctly instantiated and all required dependencies are assigned. If you’re using a framework, check the service container or dependency injection configuration.
3. Handle Exceptions Gracefully
Implement try-catch blocks to handle exceptions that could leave objects in an uninitialized state.
- Example in PHP:phpCopy code
try { $object = createObject(); $parentId = $object->getCollectionParentId(); } catch (Exception $e) { error_log($e->getMessage()); echo "Failed to retrieve parent ID."; }
4. Debug Asynchronous Code
For asynchronous operations, ensure that the object is initialized before accessing it. Using tools like promises or callbacks can help.
Preventive Measures
1. Implement Logging
Introduce logging at critical points in your application to trace object states. For instance, log the value of the object before calling the method.
2. Use Debuggers
Modern IDEs offer debugging tools that allow you to step through code and examine variables. These tools can quickly pinpoint where the null value originates.
3. Write Unit Tests
Unit tests help identify edge cases where the object might be null. Testing your code ensures stability and reduces runtime errors.
4. Collaborate on Code Reviews
Peer reviews can uncover issues that might not be immediately apparent. A fresh set of eyes on the code can often catch potential errors.
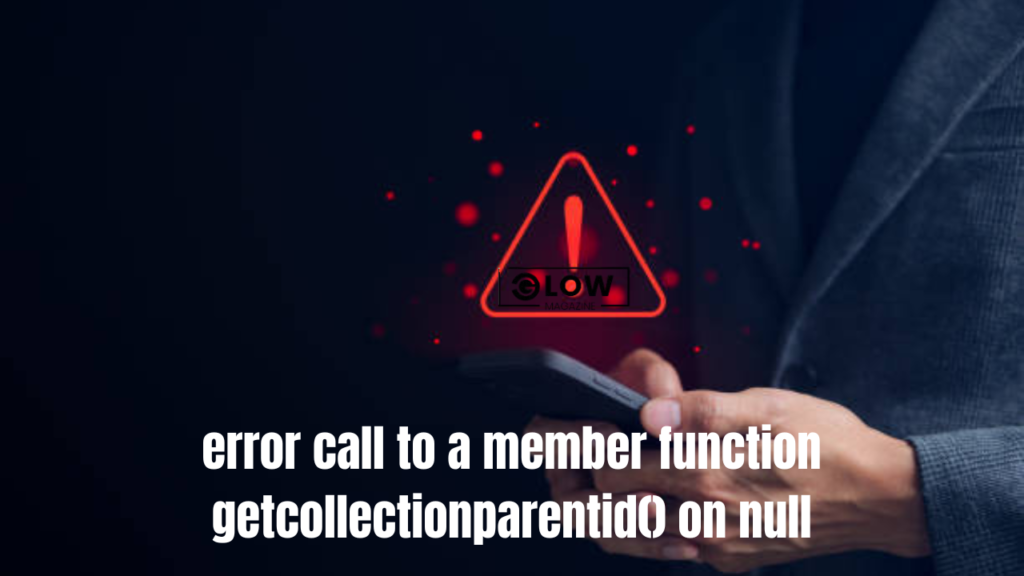
Practical Example
Let’s consider a practical example where this error might occur in a content management system (CMS):
- Problem: You attempt to retrieve the parent category of a collection, but the collection object is null.
- Code Sample:phpCopy code
$collection = getCollectionById($id); // This function returns null if the ID is invalid $parentId = $collection->getCollectionParentId(); // Error occurs here
- Solution:phpCopy code
$collection = getCollectionById($id); if ($collection === null) { echo "Invalid collection ID."; } else { $parentId = $collection->getCollectionParentId(); echo "Parent ID: " . $parentId; }
Also read: Healthtdy.xyz: Your Gateway to a Healthier Lifestyle
Final Thoughts
The “error call to a member function getCollectionParentId() on null” is a common yet manageable issue in software development. By understanding its causes and following best practices for debugging and error handling, developers can resolve this error efficiently. Proactive measures, such as null checks, robust testing, and clear object initialization, will help minimize the occurrence of this problem in future projects.
Proper coding discipline and vigilant debugging will transform this error from a frustrating obstacle into an opportunity to strengthen your programming skills.